When working with PyTorch and transformer models such as BERT, users might occasionally encounter warnings or errors related to library initialization and weight loading. Two common issues include the UserWarning: Failed to initialize NumPy: _ARRAY_API not found and problems with BERT model weight initialization. Addressing these issues is crucial to ensuring smooth execution of deep learning workflows.
Understanding the NumPy Initialization Warning
The warning “Failed to initialize NumPy: _ARRAY_API not found” may appear when importing PyTorch or working with tensor operations. This issue is typically caused by an incomplete or incompatible installation of NumPy. PyTorch relies on NumPy for certain backend functionalities, and if NumPy fails to initialize properly, users may experience unexpected behavior or performance degradation.
Possible Reasons for the Warning
- Incomplete or Corrupted NumPy Installation: If NumPy was not installed correctly or became corrupted, PyTorch may fail to interface with it.
- Version Incompatibility: PyTorch and NumPy versions may be incompatible, leading to missing symbols or API changes preventing proper initialization.
- Conflicting Packages: Other installed packages that depend on NumPy might interfere with its correct functioning.
How to Fix the Issue
To resolve this warning, consider the following steps:
- Ensure NumPy is installed by running:
pip install --upgrade numpy
- Check for any conflicting installations by using:
pip list | grep numpy
- Reinstall NumPy from scratch:
pip uninstall numpy pip install numpy
- Ensure compatibility between PyTorch and NumPy by checking their official documentation for the recommended versions.
After applying these fixes, restart your development environment (such as Jupyter Notebook or Python script) and check whether the issue persists.
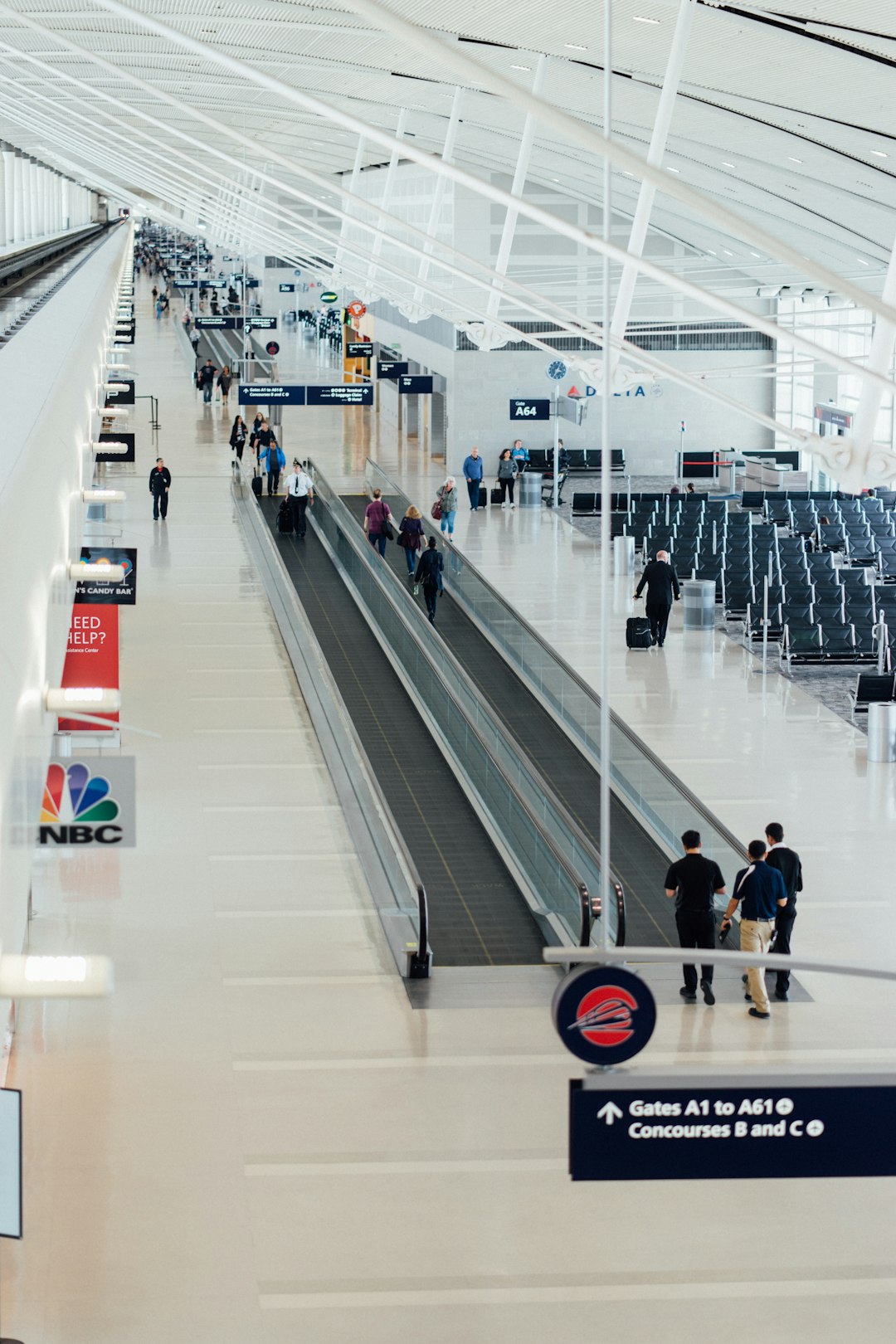
BERT Model Weight Initialization Issue
Another common issue arises when working with transformer models like BERT in PyTorch: improper weight initialization. This can lead to unstable training, poor model convergence, or suboptimal predictions.
Understanding Weight Initialization
Models like BERT come pre-trained with carefully initialized weights. When loading a pre-trained model via `transformers.BERTModel.from_pretrained(…)`, any issues related to weight loading can significantly affect performance.
Common Weight Initialization Issues
- Checkpoint Mismatch: Using a model checkpoint incompatible with the expected architecture may result in incorrect parameter loading.
- Layer Misalignment: When fine-tuning BERT, ensuring all layers initialize correctly is crucial. Missing layers can cause training instability.
- Hardcoded Initializations: If manual weight initialization is performed without care, it might override pre-trained weights, leading to suboptimal performance.
Resolving BERT Weight Initialization Problems
Here are steps to address initialization issues:
- Ensure you are using the correct model name:
from transformers import BertModel model = BertModel.from_pretrained("bert-base-uncased")
- Verify that all weights are correctly loaded by printing model parameters:
- If unexpected behavior occurs, consider reloading the model using:
model = BertModel.from_pretrained("bert-base-uncased", ignore_mismatched_sizes=True)
- When fine-tuning, always ensure gradient updates are enabled on all trainable parameters.
for name, param in model.named_parameters(): print(name, param.shape)
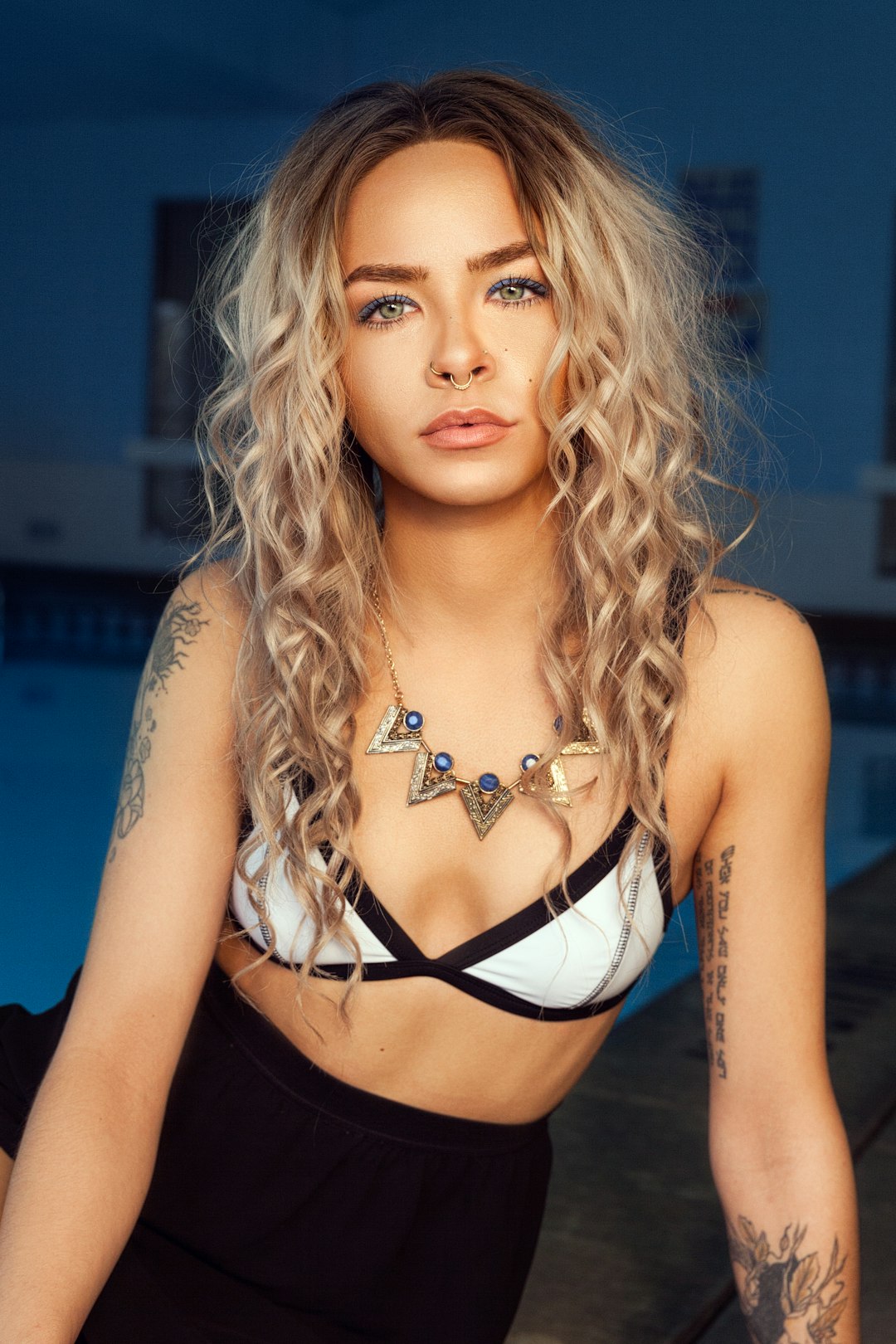
Conclusion
Both the NumPy initialization warning and BERT weight loading issues can disrupt deep learning workflows. By understanding the root causes and applying the appropriate fixes, developers can ensure a stable environment for training and inference. Keeping software versions updated, verifying dependencies, and properly handling pre-trained model weights are key strategies in avoiding these common pitfalls.